After creating some scripts to build an Azure and AVD environment, it’s time to give Intune some attention.
For this one, I will be using Powershell, POSHGUI and the excellent Intune Backup and Restore script
UPDATE: Now available on Powershell Gallery
Install-Script -Name BuildIntuneEnvironment
As usual, all scripts are freely available on GitHub, feel free to amend as much as needed:
GUI
Settings
Let’s start with the Powershell script
As with my other scripts, you get a GUI to work with which will prompt for some details which are then added into the powershell scripts prior to restore
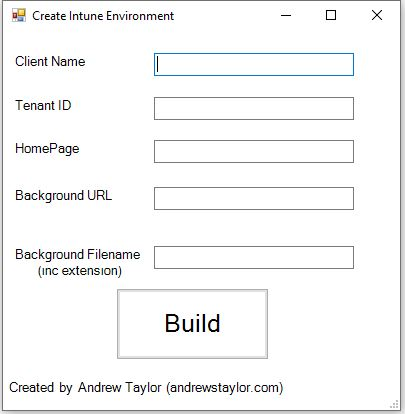
First thing we need to do is make sure the modules are installed. For this we will need Microsoft Graph to connect to Intune and the backup and restore script to do the hard work:
Write-Host "Installing Intune modules if required (current user scope)"
#Install MS Graph if not available
if (Get-Module -ListAvailable -Name Microsoft.Graph.Intune) {
Write-Host "Microsoft Graph Already Installed"
}
else {
try {
Install-Module -Name Microsoft.Graph.Intune -Scope CurrentUser -Repository PSGallery -Force
}
catch [Exception] {
$_.message
exit
}
}
#Install MS Intune Backup and Restore if not available
if (Get-Module -ListAvailable -Name IntuneBackupAndRestore) {
Write-Host "Intune Backup and Restore Already Installed"
}
else {
try {
Install-Module -Name IntuneBackupAndRestore -Scope CurrentUser -Repository PSGallery -Force
}
catch [Exception] {
$_.message
exit
}
}
#Importing Modules
Import-Module IntuneBackupAndRestore
Import-Module Microsoft.Graph.Intune
Then we need to connect to Graph and create a temp folder to store the files. We’ll also declare the variable to pass to other modules
Connect-MSGraph
#Create path for files
$DirectoryToCreate = "c:\temp"
if (-not (Test-Path -LiteralPath $DirectoryToCreate)) {
try {
New-Item -Path $DirectoryToCreate -ItemType Directory -ErrorAction Stop | Out-Null #-Force
}
catch {
Write-Error -Message "Unable to create directory '$DirectoryToCreate'. Error was: $_" -ErrorAction Stop
}
"Successfully created directory '$DirectoryToCreate'."
}
else {
"Directory already existed"
}
$random = Get-Random -Maximum 1000
$random = $random.ToString()
$date =get-date -format yyMMddmmss
$date = $date.ToString()
$path2 = $random + "-" + $date
$path = "c:\temp\" + $path2 + "\"
New-Item -ItemType Directory -Path $path
$pathvar = [PSCustomObject]@{value=$path}
Write-Host "Directory Created"
Now we download the files and remove the zip when finished to keep it clean
#Set Paths
$url = "https://github.com/andrew-s-taylor/Intune-Config/archive/main.zip"
$pathaz = "c:\temp\" + $path2 + "\Intune-Config"
$output = "c:\temp\" + $path2 + "\main.zip"
#Download Files
Invoke-WebRequest -Uri $url -OutFile $output -Method Get
Expand-Archive $output -DestinationPath $path -Force
#Remove Zip file downloaded
remove-item $output -Force
Next step is to populate the Powershell scripts with the details grabbed
$devicescript = $path + "\Intune-Config-main\Device Management Scripts\Script Content\Device Config.ps1"
#Update Client Name
(Get-Content -path $devicescript -Raw ) `
-replace '<CLIENTREPLACENAME>',$clientnameout | Set-Content -Path $devicescript
#Update O365 Tenant
(Get-Content -path $devicescript -Raw ) `
-replace '<CLIENTTENANT>',$tenantidout | Set-Content -Path $devicescript
#Update Client Homepage
(Get-Content -path $devicescript -Raw ) `
-replace '<CLIENTHOMEPAGE>',$homepageout | Set-Content -Path $devicescript
#Update Background location
(Get-Content -path $devicescript -Raw ) `
-replace '<BACKGROUNDBLOBURL>',$bloburl | Set-Content -Path $devicescript
#Update Background Name
(Get-Content -path $devicescript -Raw ) `
-replace '<BACKGROUNDFILENAME>',$backgroundfilename | Set-Content -Path $devicescript
##User Script
$userscript = $path + "\Intune-Config-main\Device Management Scripts\Script Content\User-Config.ps1"
(Get-Content -path $userscript -Raw ) `
-replace '<BACKGROUNDFILENAME>',$backgroundfilename | Set-Content -Path $userscript
Then the most important step, restore the environment
##Restore
start-IntuneRestoreConfig -Path $path
Now onto the contents of the environment
Settings Catalog:
- Device Config Policy – This is basically empty for you to populate with whatever is required from the on-prem GPOs. It is using the new Settings Catalog feature
- Office Broad Ring – Semi-Annual Enterprise channel for the majority of users
- Office Pilot Ring – Monthly Channel for your app testers
- Office Preview Ring – Current Channel for your bleeding edge users (usually IT staff)
- Office VIP Ring – Semi-Annual Targeted for any machines which must have a particular version (we all know they exist!)
Admin Template:
- Office Known Folder Move includes
- Silent sign-in
- Exclude .lnk files (to avoid those annoying multiple MS Teams icons on the desktop when logging into 2 machines)
- Silent file move
- Hide Deleted Files reminder
- Prevent users from moving their known folders
- Use OneDrive Files on Demand
Compliance policy – require everything, encryption, AV, firewall etc. I prefer to start harsh and then ease if needed
Scripts:
- Backup Profile – I’ll be blogging about this separately
- Built-in app removal Script – This is the one that comes with Quick Start. I prefer the bloat one, but this way you can pick
- Device Config – Sets the chrome homepage, configures OneDrive, allows printer installs, disables fastboot and copies a background file
- Remove Bloat – A tweaked version of Win10 Debloater to run silently
- User Config – Enables ADAL for Onedrive, unpins the MS store and sets the background for the user
Update Rings
- Broad: Semi-Annual channel – Defer feature and quality updates for 10 days each. This is for the majority of staff
- Pilot: Insider Slow – Don’t defer either. This is for your departmental app testers to try out new builds before they hit Broad
- Preview: Insider Fast – Don’t defer. This is for IT staff to test the latest and greatest
- VIP: Semi-Annual. Defer feature updates for 180 days and quality for 30. This is for your mission critical machines.
Endpoint Security
- AV policy
- Encryption policy
- Firewall Policy
- Endpoint detection policy
- Edge Security Baseline
- Windows Security baseline. This has been carefully amended so no settings are duplicated from others to avoid a clash.
Apps to get you started
- 7-zip in win32 format – MSI version so install, uninstall and detection will pre-populate
- Notepad++ in msix format (with cert)
That should then give an Intune environment which can be tailored accordingly, but without all of the initial work creating base policies